How To Make A Set A List In Python
Python lists are one of the nigh versatile data types that allow united states of america to piece of work with multiple elements at once. For case,
# a list of programming languages ['Python', 'C++', 'JavaScript']
Create Python Lists
In Python, a list is created by placing elements inside foursquare brackets []
, separated past commas.
# list of integers my_list = [ane, two, 3]
A list can take any number of items and they may exist of dissimilar types (integer, bladder, string, etc.).
# empty list my_list = [] # list with mixed data types my_list = [1, "Howdy", iii.4]
A list tin too take another listing equally an item. This is chosen a nested list.
# nested list my_list = ["mouse", [8, iv, vi], ['a']]
Access List Elements
In that location are various ways in which we can access the elements of a listing.
List Index
We can utilize the alphabetize operator []
to access an item in a listing. In Python, indices offset at 0. And then, a list having five elements volition take an index from 0 to iv.
Trying to access indexes other than these will raise an IndexError
. The index must be an integer. We can't utilize float or other types, this will effect in TypeError
.
Nested lists are accessed using nested indexing.
my_list = ['p', 'r', 'o', 'b', 'e'] # first item print(my_list[0]) # p # third item print(my_list[2]) # o # fifth detail print(my_list[four]) # eastward # Nested List n_list = ["Happy", [two, 0, ane, 5]] # Nested indexing impress(n_list[0][ane]) print(n_list[one][three]) # Error! Just integer can be used for indexing impress(my_list[4.0])
Output
p o e a five Traceback (most recent call concluding): File "<cord>", line 21, in <module> TypeError: listing indices must be integers or slices, non float
Negative indexing
Python allows negative indexing for its sequences. The alphabetize of -i refers to the last item, -2 to the 2nd terminal item and then on.
# Negative indexing in lists my_list = ['p','r','o','b','due east'] # concluding detail print(my_list[-1]) # fifth last item impress(my_list[-v])
Output
eastward p
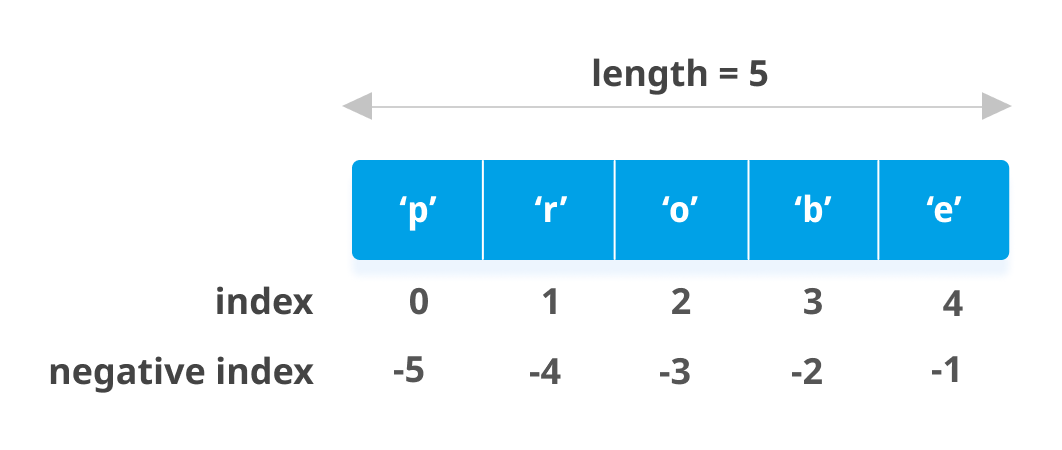
List Slicing in Python
We can access a range of items in a list past using the slicing operator :
.
# List slicing in Python my_list = ['p','r','o','g','r','a','m','i','z'] # elements from index ii to index iv print(my_list[2:5]) # elements from alphabetize 5 to finish print(my_list[5:]) # elements offset to end print(my_list[:])
Output
['o', 'g', 'r'] ['a', 'thou', 'i', 'z'] ['p', 'r', 'o', 'k', 'r', 'a', 'yard', 'i', 'z']
Note: When nosotros slice lists, the start index is inclusive but the end alphabetize is exclusive. For case, my_list[2: 5]
returns a list with elements at index 2, iii and iv, but not 5.
Add/Change Listing Elements
Lists are mutable, meaning their elements can be inverse different string or tuple.
We tin utilise the consignment operator =
to modify an item or a range of items.
# Correcting mistake values in a list odd = [2, 4, 6, 8] # change the 1st item odd[0] = 1 print(odd) # modify second to 4th items odd[one:4] = [3, 5, 7] print(odd)
Output
[1, 4, 6, eight] [i, 3, 5, 7]
Nosotros tin can add one item to a list using the suspend()
method or add together several items using the extend()
method.
# Appending and Extending lists in Python odd = [1, 3, 5] odd.suspend(vii) print(odd) odd.extend([9, eleven, xiii]) print(odd)
Output
[1, 3, 5, vii] [1, 3, 5, seven, 9, 11, 13]
We can as well employ +
operator to combine two lists. This is also called concatenation.
The *
operator repeats a list for the given number of times.
# Concatenating and repeating lists odd = [i, 3, 5] print(odd + [nine, 7, 5]) impress(["re"] * iii)
Output
[1, 3, 5, ix, vii, 5] ['re', 're', 're']
Furthermore, we tin can insert i particular at a desired location by using the method insert()
or insert multiple items by squeezing it into an empty slice of a listing.
# Sit-in of list insert() method odd = [1, 9] odd.insert(1,3) print(odd) odd[2:ii] = [5, 7] impress(odd)
Output
[ane, 3, 9] [1, 3, v, vii, 9]
Delete List Elements
We can delete one or more items from a list using the Python del argument. It tin even delete the listing entirely.
# Deleting list items my_list = ['p', 'r', 'o', 'b', 'fifty', 'e', 'g'] # delete one particular del my_list[2] print(my_list) # delete multiple items del my_list[one:five] impress(my_list) # delete the entire list del my_list # Fault: List not divers print(my_list)
Output
['p', 'r', 'b', 'fifty', 'eastward', 'm'] ['p', 'm'] Traceback (most recent telephone call last): File "<string>", line eighteen, in <module> NameError: name 'my_list' is not defined
We can apply remove()
to remove the given item or pop()
to remove an item at the given index.
The pop()
method removes and returns the last item if the index is not provided. This helps usa implement lists equally stacks (first in, last out data structure).
And, if nosotros have to empty the whole list, we tin utilise the clear()
method.
my_list = ['p','r','o','b','50','e','chiliad'] my_list.remove('p') # Output: ['r', 'o', 'b', 'l', 'e', 'm'] impress(my_list) # Output: 'o' print(my_list.pop(1)) # Output: ['r', 'b', 'l', 'e', 'thou'] print(my_list) # Output: 'm' impress(my_list.popular()) # Output: ['r', 'b', 'l', 'e'] print(my_list) my_list.clear() # Output: [] print(my_list)
Output
['r', 'o', 'b', 'l', 'e', 'm'] o ['r', 'b', 'l', 'e', 'grand'] yard ['r', 'b', 'l', 'eastward'] []
Finally, we tin also delete items in a list past assigning an empty list to a slice of elements.
>>> my_list = ['p','r','o','b','l','e','m'] >>> my_list[ii:3] = [] >>> my_list ['p', 'r', 'b', 'l', 'e', 'thousand'] >>> my_list[2:5] = [] >>> my_list ['p', 'r', 'm']
Python Listing Methods
Python has many useful list methods that makes it really easy to work with lists. Here are some of the unremarkably used listing methods.
Methods | Descriptions |
---|---|
suspend() | adds an element to the end of the list |
extend() | adds all elements of a list to some other list |
insert() | inserts an item at the defined alphabetize |
remove() | removes an item from the list |
popular() | returns and removes an chemical element at the given index |
articulate() | removes all items from the list |
alphabetize() | returns the index of the first matched item |
count() | returns the count of the number of items passed as an argument |
sort() | sort items in a list in ascending order |
reverse() | reverse the guild of items in the list |
copy() | returns a shallow copy of the list |
# Example on Python list methods my_list = [three, 8, i, 6, eight, eight, 4] # Add 'a' to the end my_list.append('a') # Output: [3, 8, one, 6, 8, 8, iv, 'a'] print(my_list) # Index of first occurrence of 8 print(my_list.index(8)) # Output: ane # Count of 8 in the list print(my_list.count(8)) # Output: 3
Listing Comprehension: Elegant fashion to create Lists
Listing comprehension is an elegant and concise fashion to create a new listing from an existing list in Python.
A listing comprehension consists of an expression followed by for argument inside square brackets.
Here is an example to make a listing with each detail being increasing power of 2.
pow2 = [two ** x for x in range(ten)] impress(pow2)
Output
[1, 2, 4, 8, sixteen, 32, 64, 128, 256, 512]
This code is equivalent to:
pow2 = [] for x in range(10): pow2.append(ii ** x)
A list comprehension can optionally contain more for
or if statements. An optional if
statement tin filter out items for the new list. Hither are some examples.
>>> pow2 = [2 ** x for 10 in range(10) if x > 5] >>> pow2 [64, 128, 256, 512] >>> odd = [x for x in range(xx) if 10 % 2 == 1] >>> odd [ane, 3, v, vii, 9, 11, 13, fifteen, 17, nineteen] >>> [x+y for 10 in ['Python ','C '] for y in ['Language','Programming']] ['Python Linguistic communication', 'Python Programming', 'C Language', 'C Programming']
Visit Python list comprehension to learn more.
Other List Operations in Python
Listing Membership Examination
We tin test if an item exists in a list or not, using the keyword in
.
my_list = ['p', 'r', 'o', 'b', 'l', 'e', 'm'] # Output: True print('p' in my_list) # Output: False print('a' in my_list) # Output: True print('c' not in my_list)
Output
True Imitation True
Iterating Through a List
Using a for
loop we tin iterate through each particular in a list.
for fruit in ['apple','banana','mango']: print("I like",fruit)
Output
I like apple tree I similar banana I similar mango
How To Make A Set A List In Python,
Source: https://www.programiz.com/python-programming/list
Posted by: goodsonsentes.blogspot.com
0 Response to "How To Make A Set A List In Python"
Post a Comment